Public Types |
Public Member Functions |
Static Public Member Functions |
Friends |
List of all members
index_metric Class Reference
#include <index_metric.h>
Inheritance diagram for index_metric:
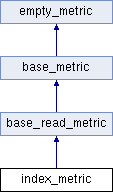
Public Types | |
enum | { TYPE = constants::Index, LATEST_VERSION = 2 } |
typedef index_metric_header | header_type |
typedef std::vector< index_info > | index_array_t |
typedef index_info | index_info_t |
typedef index_array_t::const_iterator | const_iterator |
![]() | |
typedef base_read_metric_header | header_type |
typedef constants::base_read_t | base_t |
typedef uint8_t | read_t |
![]() | |
enum | { LANE_BIT_COUNT = 6, TILE_BIT_COUNT = 26, CYCLE_BIT_COUNT = 16, READ_BIT_COUNT = 16, RESERVED_BIT_COUNT = 16, READ_BIT_SHIFT = RESERVED_BIT_COUNT, CYCLE_BIT_SHIFT = RESERVED_BIT_COUNT, TILE_BIT_SHIFT = CYCLE_BIT_SHIFT+CYCLE_BIT_COUNT, LANE_BIT_SHIFT = TILE_BIT_SHIFT+TILE_BIT_COUNT } |
typedef ::uint64_t | ulong_t |
typedef ulong_t | id_t |
typedef ::uint16_t | ushort_t |
typedef ::uint32_t | uint_t |
typedef base_metric_header | header_type |
typedef ::uint8_t | lane_t |
typedef ::uint32_t | tile_t |
typedef constants::base_tile_t | base_t |
![]() | |
typedef ::uint32_t | id_t |
typedef ::uint32_t | uint_t |
typedef constants::base_run_t | base_t |
Public Member Functions | |
index_metric () | |
index_metric (const header_type &) | |
index_metric (uint_t lane, uint_t tile, uint_t read, const index_array_t &indices) | |
size_t | size () const |
const index_info & | indices (const size_t n) const INTEROP_THROW_SPEC((model |
const index_array_t & | indices () const |
const_iterator | begin () const |
const_iterator | end () const |
float | cluster_count () const |
float | cluster_count_pf () const |
void | set_cluster_counts (const float cluster_count, const float cluster_count_pf) |
float | percent_demultiplexed (const std::string &sample_id) const |
![]() | |
base_read_metric (const uint_t lane, const uint_t tile, const uint_t read) | |
void | set_base (const uint_t lane, const uint_t tile) |
void | set_base (const uint_t lane, const uint_t tile, const uint_t read) |
template<class BaseReadMetric > | |
void | set_base (const BaseReadMetric &base) |
uint_t | read () const |
id_t | id () const |
bool | operator< (const base_read_metric &metric2) const |
![]() | |
base_metric (const uint_t lane=0, const uint_t tile=0) | |
void | set_base (const uint_t lane, const uint_t tile) |
template<class BaseMetric > | |
void | set_base (const BaseMetric &base) |
id_t | id () const |
id_t | tile_hash () const |
uint_t | lane () const |
uint_t | tile () const |
uint_t | number (const illumina::interop::constants::tile_naming_method) const |
uint_t | section (const illumina::interop::constants::tile_naming_method method) const |
uint_t | surface (const illumina::interop::constants::tile_naming_method method) const |
uint_t | swath (const illumina::interop::constants::tile_naming_method method) const |
uint_t | phyiscalLocationIndex (const illumina::interop::constants::tile_naming_method method, const uint_t section_per_lane, const uint_t tile_count, const uint_t swath_count, const bool all_surfaces) const |
uint_t | phyiscalLocationColumn (const illumina::interop::constants::tile_naming_method method, const uint_t swath_count, const bool all_surfaces) const |
uint_t | phyiscalLocationRow (const illumina::interop::constants::tile_naming_method method, const uint_t section_per_lane, const uint_t tile_count) const |
size_t | physical_location_index (const illumina::interop::constants::tile_naming_method method, const uint_t section_per_lane, const uint_t tile_count, const uint_t swath_count, const bool all_surfaces) const |
uint_t | physical_location_column (const illumina::interop::constants::tile_naming_method method, const uint_t swath_count, const bool all_surfaces) const |
uint_t | physical_location_row (const illumina::interop::constants::tile_naming_method method, const uint_t section_per_lane, const uint_t tile_count) const |
bool | operator< (const base_metric &metric2) const |
![]() | |
template<class BaseMetric > | |
void | set_base (const BaseMetric &) |
void | set_base (const uint_t, const uint_t) |
bool | operator< (const empty_metric &) const |
Static Public Member Functions | |
static const char * | prefix () |
![]() | |
static id_t | create_id (const id_t lane, const id_t tile, const id_t read) |
static id_t | read_from_id (const id_t id) |
![]() | |
static id_t | create_id (const id_t lane, const id_t tile, const id_t=0) |
static id_t | lane_from_id (const id_t id) |
static id_t | tile_hash_from_id (const id_t id) |
static id_t | tile_from_id (const id_t id) |
static const char * | suffix () |
![]() | |
static const char * | suffix () |
static id_t | create_id (const id_t, const id_t, const id_t=0) |
Friends | |
template<class MetricType , int Version> | |
struct | io::generic_layout |
Detailed Description
Index metric
The index metric holds string information describing the indexes used in the run.
- Note
- Supported versions: 1 and 2
- Test:
Confirm version 1 of the metric can be written to and read from a stream
Confirm version 1 of the metric matches known binary file
Member Typedef Documentation
typedef index_array_t::const_iterator const_iterator |
Define a constant iterator
typedef index_metric_header header_type |
Index metric header
typedef std::vector<index_info> index_array_t |
Define a index array using an underlying vector
typedef index_info index_info_t |
Define index info type
Member Enumeration Documentation
anonymous enum |
Constructor & Destructor Documentation
|
inline |
Constructor
|
inline |
Constructor
|
inline |
Constructor
- Parameters
-
lane lane number tile tile number read read number indices array of indexes
Member Function Documentation
|
inline |
Get iterator to start of indices
- Returns
- iterator to start of indices
|
inline |
Number of clusters for each tile
- Note
- Derived from tile_metric using
populate_indices
inrun_metrics::finalize_after_load
- Returns
- number of clusters
|
inline |
Number of clusters passing filter for each tile
- Note
- Derived from tile_metric using
populate_indices
inrun_metrics::finalize_after_load
- Returns
- number of clusters passing filter
|
inline |
Get iterator to end of indices
- Returns
- iterator to end of indices
|
inline |
Percentage of PF clusters on the tile that have been demultiplexed
Dependent on tile_metric
- Returns
- percent of demultiplexed PF clusters
|
inlinestatic |
Get the prefix of the InterOp filename
- Returns
- "Index"
|
inline |
Set the cluster counds dervied from tile_metric
- Parameters
-
cluster_count number of clusters cluster_count_pf number of PF clusters
Friends And Related Function Documentation
|
friend |
The documentation for this class was generated from the following file:
- /io/interop/model/metrics/index_metric.h