#include <base_metric.h>
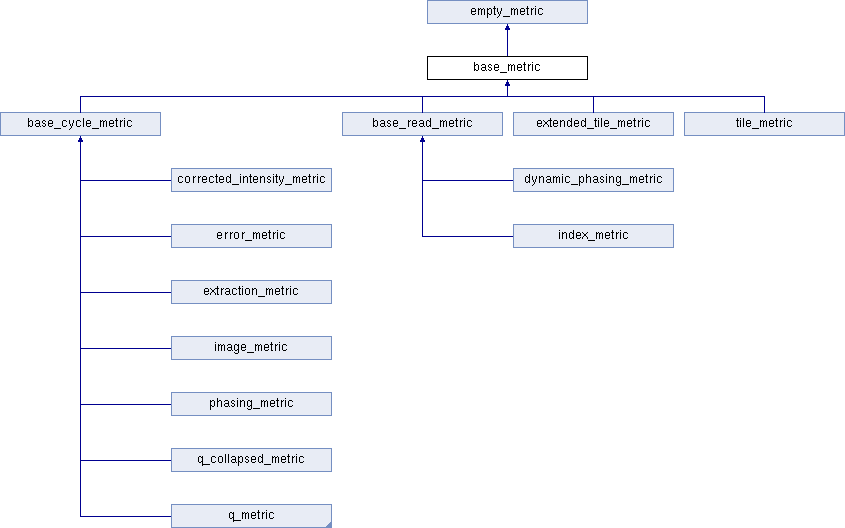
Public Types | |
enum | { LANE_BIT_COUNT = 6, TILE_BIT_COUNT = 26, CYCLE_BIT_COUNT = 16, READ_BIT_COUNT = 16, RESERVED_BIT_COUNT = 16, READ_BIT_SHIFT = RESERVED_BIT_COUNT, CYCLE_BIT_SHIFT = RESERVED_BIT_COUNT, TILE_BIT_SHIFT = CYCLE_BIT_SHIFT+CYCLE_BIT_COUNT, LANE_BIT_SHIFT = TILE_BIT_SHIFT+TILE_BIT_COUNT } |
typedef ::uint64_t | ulong_t |
typedef ulong_t | id_t |
typedef ::uint16_t | ushort_t |
typedef ::uint32_t | uint_t |
typedef base_metric_header | header_type |
typedef ::uint8_t | lane_t |
typedef ::uint32_t | tile_t |
typedef constants::base_tile_t | base_t |
![]() | |
typedef ::uint32_t | id_t |
typedef ::uint32_t | uint_t |
typedef constants::base_run_t | base_t |
Static Public Member Functions | |
static id_t | create_id (const id_t lane, const id_t tile, const id_t=0) |
static id_t | lane_from_id (const id_t id) |
static id_t | tile_hash_from_id (const id_t id) |
static id_t | tile_from_id (const id_t id) |
static const char * | suffix () |
![]() | |
static const char * | suffix () |
static id_t | create_id (const id_t, const id_t, const id_t=0) |
Detailed Description
Base class for InterOp classes that contain tile specific metrics
These classes define both a lane and tile identifier.
Member Typedef Documentation
typedef constants::base_tile_t base_t |
Define the base type
typedef base_metric_header header_type |
Base metric header that adds no functionality
typedef ::uint8_t lane_t |
Define lane type
typedef ::uint32_t tile_t |
Define tile type
typedef ::uint32_t uint_t |
Unsigned int
typedef ::uint64_t ulong_t |
Unsigned long
typedef ::uint16_t ushort_t |
Unsigned int16_t
Member Enumeration Documentation
anonymous enum |
Constructor & Destructor Documentation
|
inline |
Constructor
- Parameters
-
lane lane number tile tile number
Member Function Documentation
Unique id created from both the lane and tile
- Parameters
-
lane lane number tile tile number
- Returns
- unique id
|
inline |
Unique id created from both the lane and tile
- Returns
- unique identifier
|
inline |
Lane number
- Returns
- lane number
Get the lane from the unique lane/tile id
- Parameters
-
id unique lane/tile id
- Returns
- lane number
|
inline |
Tile number
Calculates the number of the tile from the tile id.
- Returns
- number of the tile.
|
inline |
Comparison operator used to sort the entries in order of their IDs
- Parameters
-
metric2 metric to compare with the current object
- Returns
- true if this object's ID is less than metric2's ID
|
inline |
Column of the physical location of tile within the flowcell
- Deprecated:
- Will be removed in 1.1.x (use phyiscal_location_column instead)
- Parameters
-
method the tile naming method swath_count number of swaths all_surfaces layout all surfaces of the flowcell
- Returns
- column of the physical location within the flowcell
|
inline |
Index of the physical location of tile within the flowcell
- Deprecated:
- Will be removed in 1.1.x (use physical_location_index instead)
- Parameters
-
method the tile naming method section_per_lane number of sections per lane tile_count number of tiles swath_count number of swaths all_surfaces layout all surfaces of the flowcell
- Returns
- index of the physical location within the flowcell
|
inline |
Row of the physical location of tile within the flowcell
- Deprecated:
- Will be removed in 1.1.x (use phyiscal_location_row instead)
- Parameters
-
method the tile naming method section_per_lane number of sections per lane (number of cameras that cover the lane) tile_count number of tiles
- Returns
- row of the physical location within the flowcell
|
inline |
Column of the physical location of tile within the flowcell
- Parameters
-
method the tile naming method swath_count number of swaths all_surfaces layout all surfaces of the flowcell
- Returns
- column of the physical location within the flowcell
|
inline |
Index of the physical location of tile within the flowcell
- Parameters
-
method the tile naming method section_per_lane number of sections per lane (number of cameras that cover the lane) tile_count number of tiles swath_count number of swaths all_surfaces layout all surfaces of the flowcell
- Returns
- index of the physical location within the flowcell
|
inline |
Row of the physical location of tile within the flowcell
- Parameters
-
method the tile naming method section_per_lane number of sections per lane tile_count number of tiles
- Returns
- row of the physical location within the flowcell
|
inline |
Section number
Calculates the section of the tile from the tile id.
- Returns
- section of the tile.
Set id
- Parameters
-
lane lane number tile tile number
|
inline |
Set the base metric identifiers
- Parameters
-
base layout base
|
inlinestatic |
Get the metric name suffix
- Returns
- empty string
|
inline |
Surface number
Calculates the surface of the tile from the tile id.
- Returns
- surface of the tile.
|
inline |
Swath number
Calculates the surface of the tile from the tile id.
- Returns
- surface of the tile.
|
inline |
Tile id
The tile id can have either a 4-digit format or a 5-digit format.
4-digit: 1234 -> surface (1) swath (2) tile_number (34)
5-digit: 12345 -> surface (1) swath (2) section(3) tile_number (45)
- Returns
- tile number
Get the tile hash from the unique lane/tile/cycle id
- Parameters
-
id unique lane/tile/cycle id
- Returns
- tile hash number
|
inline |
Unique id created from both the lane and tile
- Returns
- unique identifier
Get the tile hash from the unique lane/tile/cycle id
- Parameters
-
id unique lane/tile/cycle id
- Returns
- tile hash number
The documentation for this class was generated from the following file:
- /io/interop/model/metric_base/base_metric.h