Public Types |
Public Member Functions |
Static Public Member Functions |
Friends |
List of all members
extended_tile_metric Class Reference
#include <extended_tile_metric.h>
Inheritance diagram for extended_tile_metric:
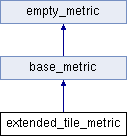
Public Types | |
enum | { TYPE = constants::ExtendedTile, LATEST_VERSION = 3 } |
![]() | |
enum | { LANE_BIT_COUNT = 6, TILE_BIT_COUNT = 26, CYCLE_BIT_COUNT = 16, READ_BIT_COUNT = 16, RESERVED_BIT_COUNT = 16, READ_BIT_SHIFT = RESERVED_BIT_COUNT, CYCLE_BIT_SHIFT = RESERVED_BIT_COUNT, TILE_BIT_SHIFT = CYCLE_BIT_SHIFT+CYCLE_BIT_COUNT, LANE_BIT_SHIFT = TILE_BIT_SHIFT+TILE_BIT_COUNT } |
typedef ::uint64_t | ulong_t |
typedef ulong_t | id_t |
typedef ::uint16_t | ushort_t |
typedef ::uint32_t | uint_t |
typedef base_metric_header | header_type |
typedef ::uint8_t | lane_t |
typedef ::uint32_t | tile_t |
typedef constants::base_tile_t | base_t |
![]() | |
typedef ::uint32_t | id_t |
typedef ::uint32_t | uint_t |
typedef constants::base_run_t | base_t |
Static Public Member Functions | |
static const char * | prefix () |
![]() | |
static id_t | create_id (const id_t lane, const id_t tile, const id_t=0) |
static id_t | lane_from_id (const id_t id) |
static id_t | tile_hash_from_id (const id_t id) |
static id_t | tile_from_id (const id_t id) |
static const char * | suffix () |
![]() | |
static const char * | suffix () |
static id_t | create_id (const id_t, const id_t, const id_t=0) |
Friends | |
template<class MetricType , int Version> | |
struct | io::generic_layout |
Detailed Description
Extended tile metric
The extended tile metric reports tile metrics such as the occupancy aka number of empty wells on a tile.
- Note
- Supported versions: 2 and 3
- Test:
Confirm version 1 of the metric can be written to and read from a stream
Confirm version 1 of the metric matches known binary file
Member Enumeration Documentation
anonymous enum |
Constructor & Destructor Documentation
|
inline |
Constructor
|
inline |
Constructor
|
inline |
Constructor
- Parameters
-
lane lane number tile tile number occupied number of occupied wells upper_left coordinate of upper left fiducial
Member Function Documentation
|
inlinestatic |
Get the prefix of the InterOp filename
- Returns
- "ExtendedTile"
|
inline |
Setter
- Note
- Version 1 and 2
- Parameters
-
lane lane number tile tile number occupied number of occupied wells upper_left coordinate of upper left fiducial
|
inline |
Set the cluster count in kilo bases
Derived from tile_metric using populate_percent_occupied
in run_metrics::finalize_after_load
- Parameters
-
cluster_count_k cluster count in kilo bases
Friends And Related Function Documentation
|
friend |
The documentation for this class was generated from the following file:
- /io/interop/model/metrics/extended_tile_metric.h