#include <metric_set.h>
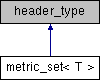
Public Types | |
enum | { TYPE =metric_attributes<T>::TYPE, LATEST_VERSION =metric_attributes<T>::LATEST_VERSION } |
typedef std::vector< T > | metric_array_t |
typedef T::header_type | header_type |
typedef T::base_t | base_t |
typedef T | metric_type |
typedef metric_set< T > | metric_set_type |
typedef T::id_t | id_t |
typedef T::uint_t | uint_t |
typedef std::vector< uint_t > | id_vector |
typedef std::vector< id_t > | key_vector |
typedef metric_array_t::size_type | size_type |
typedef std::set< uint_t > | id_set_t |
typedef std::map< id_t, size_t > | offset_map_t |
typedef metric_comparison< T > | metric_comparison_t |
typedef metric_array_t::const_iterator | const_iterator |
typedef metric_array_t::iterator | iterator |
Public Member Functions | |
metric_set (const ::int16_t version) | |
metric_set (const header_type &header=header_type::default_header(), const ::int16_t version=0) | |
metric_set (const metric_array_t &vec, const ::int16_t version, const header_type &header) | |
void | copy_by_tile (const metric_set< T > &origin, const base_metric &tile_id) |
void | append_tiles (const metric_set< T > &origin, const base_metric &tile_id) |
bool | data_source_exists () const |
void | data_source_exists (const bool exists) |
const_iterator | begin () const |
const_iterator | end () const |
iterator | begin () |
iterator | end () |
void | sort () |
void | rebuild_index (const bool update_ids=false) |
void | resize (const size_t n) |
void | reserve (const size_t n) |
void | trim (const size_t n) |
void | insert (const metric_type &metric) |
void | insert (const id_t id, const metric_type &metric) |
void | remove (iterator &it) |
metric_type & | operator[] (const size_t n) INTEROP_THROW_SPEC((model |
const metric_type & | operator[] (const size_t n) const INTEROP_THROW_SPEC((model |
metric_type & | at (const size_t n) INTEROP_THROW_SPEC((model |
const metric_type & | at (const size_t n) const INTEROP_THROW_SPEC((model |
void | set_version (const ::int16_t version) |
key_vector | keys () const |
id_vector | lanes () const |
size_t | lane_count () const |
size_t | max_lane () const |
id_vector | tile_numbers_for_lane (const uint_t lane) const |
void | populate_tile_numbers_for_lane (id_set_t &tile_number_set, const uint_t lane) const |
void | populate_tile_numbers_for_lane_surface (id_set_t &tile_number_set, const uint_t lane, const uint_t surface, const constants::tile_naming_method naming_convention) const |
id_vector | tile_numbers () const |
metric_array_t | metrics_for_lane (const uint_t lane) const |
void | metrics_for_lane (metric_array_t &lane_metrics, const uint_t lane) const |
id_vector | cycles () const |
metric_array_t | metrics_for_cycle (const uint_t cycle) const |
size_t | size () const |
bool | empty () const |
::int16_t | version () const |
void | clear () |
const metric_array_t & | metrics () const |
offset_map_t & | offset_map () |
metric_type & | get_metric_ref (uint_t lane, uint_t tile, uint_t cycle=0) INTEROP_THROW_SPEC((model |
metric_type & | get_metric_ref (id_t key) INTEROP_THROW_SPEC((model |
size_t | find (const uint_t lane, const uint_t tile, const uint_t cycle=0) const |
size_t | find (const id_t id) const |
const metric_type & | get_metric (const uint_t lane, const uint_t tile, const uint_t cycle=0) const INTEROP_THROW_SPEC((model |
bool | has_metric (const uint_t lane, const uint_t tile, const uint_t cycle=0) const |
const metric_type & | get_metric (const id_t key) const INTEROP_THROW_SPEC((model |
bool | has_metric (const id_t id) const |
void | clear_lookup () |
Static Public Member Functions | |
static const char * | prefix () |
static const char * | suffix () |
Protected Attributes | |
metric_array_t | m_data |
::int16_t | m_version |
bool | m_data_source_exists |
offset_map_t | m_id_map |
Detailed Description
template<typename T>
class illumina::interop::model::metric_base::metric_set< T >
Metric set holds a collection metrics
This class holds a map that maps a unique id to the metric.
Member Typedef Documentation
typedef T::base_t base_t |
Define the base type for the metric
typedef metric_array_t::const_iterator const_iterator |
Const metric iterator
typedef T::header_type header_type |
Define a write header
typedef T::id_t id_t |
Define a ID type
typedef metric_array_t::iterator iterator |
Metric iterator
typedef std::vector<id_t> key_vector |
Define an key vector
typedef std::vector<T> metric_array_t |
Define a collection of metrics
typedef metric_comparison<T> metric_comparison_t |
Define a safe comparison for ids
typedef metric_set<T> metric_set_type |
Define a metric set type
typedef T metric_type |
Define a metric type
typedef std::map<id_t, size_t> offset_map_t |
Define offset map
typedef metric_array_t::size_type size_type |
Define the size type
typedef T::uint_t uint_t |
Define a lane/tile/cycle id type
Member Enumeration Documentation
Constructor & Destructor Documentation
|
inline |
Constructor
- Parameters
-
version version of the file format
|
inline |
Constructor
- Parameters
-
header header information for the metric set version version of the file format
|
inline |
Constructor
- Parameters
-
vec array of metrics version of the metric format header header information for the metric set
Member Function Documentation
|
inline |
Adds records only for a specific tile
- Parameters
-
origin full metric set tile_id selected tile
|
inline |
Get a metric at the given index
- Deprecated:
- Will be removed in next feature version (use operator[] instead for C++ Code)
- Parameters
-
n index
- Returns
- metric
|
inline |
Get a metric at the given index
- Deprecated:
- Will be removed in next feature version (use operator[] instead for C++ Code)
- Parameters
-
n index
- Returns
- metric
|
inline |
Get start of metric collection
- Returns
- iterator to start of metric collection
|
inline |
Get start of metric collection
- Returns
- iterator to start of metric collection
|
inline |
Clear the metrics in the metric set
|
inline |
Clear the lookup table.
|
inline |
Copy records only for a specific tile
- Parameters
-
origin full metric set tile_id selected tile
|
inline |
Get a list of all cycles listed in the metric set
- Note
- Returns empty array for metrics that do not have a cycle identifier
- Returns
- vector of cycle numbers
|
inline |
Flag that indicates whether the data source exists
- Returns
- true if the data source, e.g. file, exists
|
inline |
Set flag that indicates whether the data source exists
- Parameters
-
exists true if the data source, e.g. file, exists
|
inline |
Test if metric set is empty
- Returns
- true if metric set is empty
|
inline |
Get end of metric collection
- Returns
- iterator to end of metric collection
|
inline |
Get end of metric collection
- Returns
- iterator to end of metric collection
Find index of metric given the id. If not found, return number of metrics
- Parameters
-
lane lane tile tile cycle cycle
- Returns
- index of metric or number of metrics
|
inline |
Find index of metric given the id. If not found, return number of metrics
- Parameters
-
id id
- Returns
- index of metric or number of metrics
|
inline |
Get metric for lane, tile and cycle
- Parameters
-
lane lane tile tile cycle cycle
- Returns
- metric
|
inline |
Get metric for a unique identifier
- Parameters
-
key unique id built from lane, tile and cycle (if available)
- Returns
- metric
|
inline |
Get metric for lane, tile and cycle
- Parameters
-
lane lane tile tile cycle cycle
- Returns
- metric
|
inline |
Get metric for a unique identifier
- Parameters
-
key unique id built from lane, tile and cycle (if available)
- Returns
- metric
Test if set has metric
- Parameters
-
lane lane tile tile cycle cycle
|
inline |
Test if set has metric
- Parameters
-
id id
|
inline |
Add a metric to the metric set
- Parameters
-
metric metric to add to set
|
inline |
Add a metric to the metric set
- Parameters
-
id unique id for metric metric metric to add to set
|
inline |
Get a list of all available lane numbers
- Returns
- vector of lane numbers
|
inline |
Get the number of lanes in the data
- Returns
- number of lanes
|
inline |
Get a list of all available lane numbers
- Returns
- vector of lane numbers
|
inline |
Get maximum lane number
- Returns
- maximum lane number
|
inline |
Get the metrics in a vector
- Returns
- vector of metrics
|
inline |
Get a list of all available metrics for the specified cycle
- Note
- Returns empty array for Tile Metrics, which does not contain a cycle identifier
- Parameters
-
cycle cycle number
- Returns
- vector of metrics that map to the given cycle
|
inline |
Get a list of all available metrics for the specified lane
- Parameters
-
lane lane number
- Returns
- vector of tile numbers
|
inline |
Get a list of all available metrics for the specified lane
- Parameters
-
lane_metrics destination metric array lane lane number
|
inline |
Get the current id offset map
- Returns
- id offset map
|
inline |
Get a metric at the given index
- Parameters
-
n index
- Returns
- metric
|
inline |
Get a metric at the given index
- Parameters
-
n index
- Returns
- metric
Get a list of all available tile numbers for the specified lane
- Note
- this does not clear the set!
- Parameters
-
tile_number_set destination set to ensure unique tile numbers lane lane number
|
inline |
Get a list of all available tile numbers for the specified lane
- Note
- this does not clear the set!
- Parameters
-
tile_number_set destination set to ensure unique tile numbers lane lane number surface surface number naming_convention tile naming convetion enum
|
inlinestatic |
Get the prefix of the InterOp filename
- Returns
- prefix
|
inline |
Rebuild the index map and update the cycle state
- Note
- This function clears the lookup table for most metrics if update_ids is false (exceptions are Tile and DynamicPhasing
- Parameters
-
update_ids rebuild the lookup table with new ids
|
inline |
Remove a metric from the metric set
- Parameters
-
it iterator to metric to remove
|
inline |
Reserve the number of places in the metric vector
- Parameters
-
n maximum number of elements
|
inline |
Resize the number of places in the metric vector
- Parameters
-
n expected number of elements
|
inline |
Set the version of the InterOp file
The InterOp file version determines which metric fields are populated. Each metric lists which version is supported.
- Parameters
-
version version number
|
inline |
Number of metrics in the metric set
- Returns
- number of metrics
|
inline |
Sort the metric collection
|
inlinestatic |
Get the suffix of the InterOp filename
- Returns
- suffix
|
inline |
Get a list of all available tile numbers
- Returns
- vector of tile numbers
Get a list of all available tile numbers for the specified lane
- Parameters
-
lane lane number
- Returns
- vector of tile numbers
|
inline |
Trim the set to the proper number of metrics
- Parameters
-
n actual size of the metric set
|
inline |
Version of the InterOp file parsed
The InterOp file version determines which metric fields are populated. Each metric lists which version is supported.
- Returns
- interop file version
Member Data Documentation
|
protected |
Array of metric data
|
protected |
Does the file or other source exist
|
protected |
Map unique identifiers to the index of the metric
|
protected |
Version of the metric read
The documentation for this class was generated from the following file:
- /io/interop/model/metric_base/metric_set.h